【Unity】System.Action 呼び出し時の null チェックを省略する
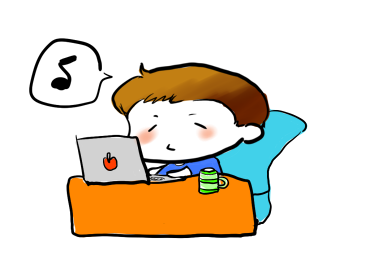
nullチェックをあちらこちらに挟みすぎるとコードの見通しが悪くなるため省略できる様にしています。
using System;
/// <summary>
/// Action拡張メソッド
/// </summary>
public static class ActionExtensions
{
/// <summary>
/// Action呼び出し。
/// </summary>
static public void Call( this Action self )
{
if(self != null)
{
self();
}
}
/// <summary>
/// Action呼び出し(引数1つ)
/// </summary>
public static void Call<T>( this Action<T> self, T arg )
{
if(self != null)
{
self(arg);
}
}
/// <summary>
/// Action呼び出し(引数2つ)
/// </summary>
public static void Call<T1, T2>( this Action<T1, T2> self, T1 arg1, T2 arg2 )
{
if(self != null)
{
self(arg1, arg2);
}
}
/// <summary>
/// Action呼び出し(引数3つ)
/// </summary>
public static void Call<T1, T2, T3>( this Action<T1, T2, T3> self, T1 arg1, T2 arg2, T3 arg3 )
{
if (self != null)
{
self(arg1, arg2, arg3);
}
}
/// <summary>
/// Func呼び出し
/// </summary>
public static TResult Call<TResult>(this Func<TResult> self, TResult result = default(TResult))
{
return self != null ? self() : result;
}
/// <summary>
/// Func呼び出し(引数1つ)
/// </summary>
public static TResult Call<T, TResult>(this Func<T, TResult> self, T arg, TResult result = default(TResult))
{
return self != null ? self(arg) : result;
}
/// <summary>
/// Func呼び出し(引数2つ)
/// </summary>
public static TResult Call<T1, T2, TResult>(this Func<T1, T2, TResult> self, T1 arg1, T2 arg2, TResult result = default(TResult))
{
return self != null ? self(arg1, arg2) : result;
}
/// <summary>
/// Func呼び出し(引数3つ)
/// </summary>
public static TResult Call<T1, T2, T3, TResult>(this Func<T1, T2, T3, TResult> self, T1 arg1, T2 arg2, T3 arg3, TResult result = default(TResult))
{
return self != null ? self(arg1, arg2, arg3) : result;
}
/// <summary>
/// 存在チェックチェック。
/// </summary>
static public bool HasAction( this Action self )
{
return (self != null) ? true : false;
}
}
使い方
System.Action action = null;
action.call(); // 個々で null チェックせずに済む。