【Unity】ParticleSystem 拡張メソッド
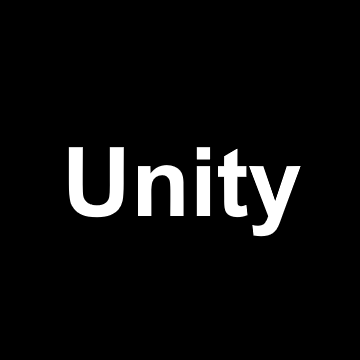
親子構造の ParticleSystem を一括で再生、停止したかったので拡張メソッドを作りました。ParticleSystem が親子になっているだけなら親子関係を作るだけで子も自動再生されるのでは?思った方もいると思いますが、今回は間に ParticleSystem の無いGameObjectが挟まる様な構造の場合でも再生したいなと思い拡張しました。
ParticleSystem の付いた GameObject
|_ParticleSystem の付いた居ない GameObject
|_ParticleSystem の付いた GameObject
こんな構成の場合を想定しています。
目次
スクリプト
using UnityEngine;
namespace mira
{
/// <summary>
/// ParticleSystem 拡張メソッド
/// </summary>
public static class ParticleSystemExtension
{
/// <summary>
/// 子を含む全てのParticleを停止します。
/// </summary>
public static void PlayAll(this ParticleSystem[] self, bool _withChildren = true)
{
Debug.Assert(self != null && self.Length > 0);
foreach (var ps in self)
{
ps.Play(_withChildren);
}
}
/// <summary>
/// 子を含む全てのパーティクルを再生します。
/// </summary>
public static void PlayAll(this ParticleSystem _self, bool _withChildren = true)
{
ParticleSystem[] systems = _self.GetComponentsInChildren<ParticleSystem>();
Debug.Assert(systems != null && systems.Length > 0);
systems.PlayAll(_withChildren);
}
/// <summary>
/// 子を含む全てのパーティクルを停止します。
/// </summary>
public static void StopAll(this ParticleSystem _self, bool _withChildren = true)
{
ParticleSystem[] systems = _self.GetComponentsInChildren<ParticleSystem>();
Debug.Assert(systems != null && systems.Length > 0);
systems.StopAll(_withChildren);
}
/// <summary>
/// 子を含む全てのParticleを停止します。
/// </summary>
public static void StopAll(this ParticleSystem[] self, bool _withChildren = true)
{
Debug.Assert(self != null && self.Length > 0);
foreach (var ps in self)
{
ps.Stop(_withChildren);
}
}
}
}
使い方
var particleSystem = gameObject.GetComponent<ParticleSystem>();
// 一括再生
particleSystem.PlayAll();
// 一括停止
particleSystem.StopAll();